- Most Important - you must have the latest update (new features and bug fixes) - see these instructions.
- Important - it is highly recommended to begin from this sample starting sketch, so all the orientations needed by the code are correct: https://a360.co/3LQxHCG
- Demo Video (screencast): https://autode.sk/3Y7hJYG
- The Chord Formula input box is a python expression which must return 1 ore more numbers in a list.
- Airfoil Tools versions greater than 1.20230217 can optionally use a dictionary instead of a list, and, can use "None" for any list positional value to use the default, and can use "Manual_Foil" and "Skip" options.
- This expression is repeatedly evaluated* many times for each section, to obtain the chord and other adjustments you want to apply to each blade section profile.
- The formula has access to a range of variables it can use (see below) to compute and return this information
- The list of numbers the formula should return is:-
[ Chord_Len, Tail_Offset, Scimitar, Hedral, X_tilt, Lift_Amount, AoA_adjust, Manual_Foil, Skip, Spline_Type, Repair ]
-or- (versions > 1.20230217)
{'Chord_Len': 0.0075, 'Tail_Offset': 0, 'Scimitar': 0, 'Hedral': 0, 'X_tilt': 0, 'Lift_Amount': 1, 'AoA_adjust': 0, 'Manual_Foil': '', 'Skip': 0 'Spline_Type':1, 'Repair':0}
Where:
- Chord_Len
- Required: the length of the chord of this blade section
- Tail_Offset
- Optional, default=0: How far to move the tail of the section forward (+) or backward (-) of the root-to-tip line. 0 places all blade tails in a straight line.
- Scimitar
- Optional, default=0: How far to advance this section ahead (+) or behind (-) the root-to-tip line. If you want to rake back your blade tips, this is the number to use
- Hedral
- Optional, default=0: How far to shift this section into (+) or away from (-) the incoming flow. Makes non-flat blades, which trace a shape like a bowl (+) or mushroom (-) as they rotate
- X_tilt
- Optional, default=0: # of degrees to tilt the section along the X axis. 90 degrees, combined with Hedral, at the tip would make winglets
- Lift_Amount
- Optional, default=1: What kind of foil shape to use: 1 is a wing (makes lift) 0 is a strut (zero lift - handy for reducing vortices, interesting to combine with Hedral and X_tilt)
- AoA_adjust
- Optional, default=0: Degrees to modify the existing ideal AoA section pitch by, handy for tip washout
- Manual_Foil
- Optional, default='': name (e.g. optbs_spline_Re500000_N4_uag8814320.dat) or full local-file-system pathname of a custom .dat file to use for this section *
- Skip
- Optional, default=False: Set to true to skip attempting to patch or loft this section (useful for sections on props that will be "inside" the hub assembly) *
- Spline_Type
- Optional, default=0: fit-point(0) or cubic(1) Manual_Foil type (when loading foils from your own .dat files) *
- Repair
- Optional, default=0: ste to 1 to run a repair and normalize when loading foils from your own .dat files *
- *
- Airfoil Tools versions greater than 1.20230217 only can use "Manual_Foil", "Skip", "Spline_Type", and "Repair" options. Use 'Manual_Foil':["C:/tmp/sect1.dat","C:/tmp/sect2.dat"][s-1] to specify the foil for each section
- Example 1:
[ (u<=0.4) * (1-u) * root_chord + (u>0.4) * (section[s-1]["speed"] / speed) * section[s-1]["chord"] ]
(The above formula is in two parts; the first applies to the 40% of the blade from the hub outwards: (u<=0.4) - which scales the chord down relative to how far away it is from the axis, the rest of the blade (from 40% to the tip: (u>0.4)) is scaled by the chord of the previous blade section relative to how fast it will be moving, which is a way to keep the reynolds number constant across most of the blade area)

- Example 2:
[ (u<=0.4) * (1-u) * root_chord + (u>0.4) * (section[s-1]["speed"] / speed) * section[s-1]["chord"] , 0 , 0 , u**6*tip_chord ]
(The above bends the tips into the wind by the length of the tip_chord line you select. Note the commas, setting Tail_Offset=0, Scimitar=0, and Hedral=u**6*tip_chord)
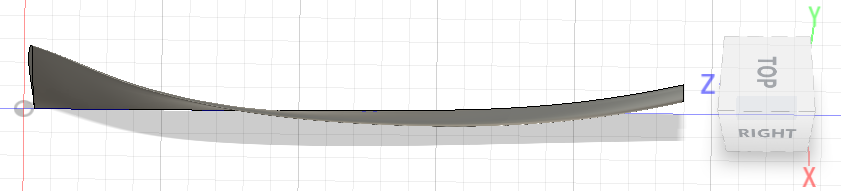
- Example 3:
[ (u<=0.4) * (1-u) * root_chord + (u>0.4) * (section[s-1]["speed"] / speed) * section[s-1]["chord"] , -chord/2 , (u>=0.9) * ((0.9-u)*10)**2 * -tip_chord , u**6*tip_chord ]
(The above centers each section on the root-to-tip line (-chord/2), rakes the tips backwards scimitar-style ((u>=0.9) * ((0.9-u)*10)**2 * -tip_chord), and bends the tips into the wind (u**6*tip_chord) )
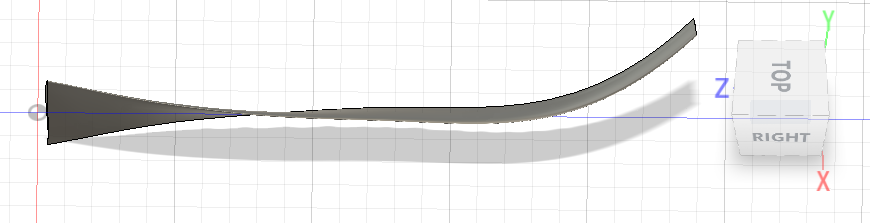
- Example 4:
[ sin(pi*( u - 0.117647059 ) * 1.117647059 )* tip_chord *(u>0.226) + (u<=0.226)* root_chord ]
(Blade section chords are a function of how long you make the tip line you select)
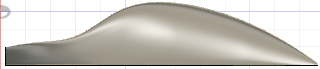
- Example 5:
[ root_chord / sin( ( zero_aoa - aoa ) / 180 * pi ) ]
(The nose and tail of all sections are in the same plane - chords are longer at the tips owing to their pitch)
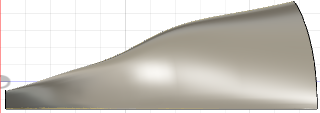
- Variables:
for s in range(0,num_profiles): # 0(hub) upto 12 (tip)
section_radius=prop_radius * (s/(num_profiles-1))**2 # tip (prop_radius) down to zero
section_speed=rps * math.pi * 2 * (section_radius) # profile rotational speed in M/S
# https://www.intechopen.com/books/advances-in-wind-power/low-speed-wind-turbine-design
# Pturb watts = .5 * efficiency(betz) * density kg/m^3 * area m^2 * speed^3 m/s (where Pturb is the mechanical power of the turbine in Watts )
if section_speed>0:
zero_aoa=math.atan((speed_loss_factor * in_speed) / section_speed ) / math.pi * 180 # Work out the needed pitch at this section for the RPM and speed (in_speed is less because of betz limit)
else:
zero_aoa=90
section_translational_speed = ( (section_speed)**2 + (speed_loss_factor * in_speed)**2 ) ** 0.5 # Work out the actual airspeed for each section
section.append({'section_radius':section_radius, # Distance of this section from the hub (e.g. tip = prop radius)
'u':(s/(num_profiles-1))**2 , # Proportion of this section away from the hub. 0= hub, 1=tip
's':s, # integer station number - best not to use, since sections are not equal spaced
'speed':section_translational_speed, # actual airspeed of this section
'zero_aoa': zero_aoa, # AoA at this station which would be directly into the flow
'rotational_speed':section_speed, # How fast is this turning here - best not to use, since the flow makes this faster (props) or slower (turbines)
'chord':0, # the output of your formula goes here - so your formula can refer to results it came up with for other sections
'foil':{}, # everything about the selected arifoil (L/D ratios etc)
'aoa':0 }) # The AoA that the best suited foil profile will assume (above the zero_aoa of course)
localok={'s':s, # the integer
'section':section, # the [list]
'diameter':prop_radius*2,
'root_chord':root_chord, # the length of the axis line you selected
'tip_chord':tip_chord, # the length of the tip line you selected. 0 if you sleected a point instead of a line for the tip
'flow_speed':in_speed, # how fast you said the flow was moving
# density, viscosity, medium, altitude, etc etc # coming soon...
'section_radius':section[s]['section_radius'], # how far this is away from the hub axis
'u':section[s]['u'], # see above
'n':num_profiles, # how many profiles you chose to have - defaults to 12 (12=tip, 0 ( never used) = hub, 1=first profile near the hub
'speed':section[s]['speed'], # see above
'zero_aoa':section[s]['zero_aoa'], # see above
'rotational_speed':section[s]['rotational_speed'], # see above
'chord':section[s]['chord'], # see above
'foil':section[s]['foil'], # see above
'aoa':section[s]['aoa'] } # see above
- If you make a mistake, detailed information about what went wrong, along with all the variables is printed in your text-commands window; be sure to turn this on in your Airfoil Tools settings and open this window in Fustion360 to see it.
- * Your expression is first evalutated for every foil section (tip to hub order), in a loop, which repeats by 1+number_of_sections (e.g. 13 times if you've got 12 sections). The last evaluation will be the result it uses. This means that you can refer to the result of your epxression as part of your expression if you need to; e.g. this is OK, and would limit the length of the chord to the maximum length of the root you picked, regardless of what the rest of your expression returns:-
[ (chord >= hub_root) * hub_root + (chord < hub_root) * ( some expression computing chord ) ]
- Hint re: logic inside expressions: this expression: (a>b) is a logic operation - it evaluates to 1 if true, and 0 if false - so if you multiply by this expression, you can include (true) or exclude (false) other parts of your expression.
If you need more instructions, leave me a note on the Forum and I'll finish writing them.
Variables from an example run:
{
'diameter' => '1.26',
's' => 12,
'num_profiles' => 12,
'aoa' => '3.3',
'flow_speed' => '6.94444444444444',
'section_radius' => '0.63',
'tip_chord' => '0',
'speed' => '177.794693242818',
'root_chord' => '0.123',
'rotational_speed' => '174.829631172272',
'zero_aoa' => '10.4785342765095',
'u' => '1',
'chord' => '0.0416832306484338',
'chord_formula' => '[ ((68-10*(( u <0.183)*( u >0.126)*(( u -0.126)/(0.126-0.183))))*( u <=0.183)+( u >0.183) * ((sin( u -0.183+1.4)-0.8)*5*39+42)) /1000, -chord/2]',
'foil' => {
'CD' => '0.00884978018634026',
'CDf' => '0.005',
'Cpmin' => '-1.36452160346992',
'CL' => '1.33965433870629',
'src' => 'retestc_7.txt',
'xver' => '6.991',
'n' => 3,
'CDp' => '0.00143473501628707',
'iver' => '1.2020052',
'clcd' => '151.377131465261',
'CDpres' => '0.00385',
'diter' => 3,
'Cm' => '-0.228915601237377',
'xy' => [
1,
0,
'0.976945036273593',
'0.00939462486134211',
'0.851587448517823',
'0.0496390744878245',
'0.698540191794841',
'0.104268363253013',
'0.518781216857077',
'0.131891298315886',
'0.327066076343497',
'0.126506814697001',
'0.150680323221142',
'0.0905868417616979',
'0.0395735702112627',
'0.0422057839630234',
0,
0,
'0.0572978334471142',
'-0.00364596812263481',
'0.162662166368623',
'0.00417792129992324',
'0.31244663898479',
'0.0140082041081467',
'0.472099215454883',
'0.0216480338049078',
'0.578263018146512',
'0.0257475498551808',
'0.759908593228447',
'0.0266643873940281',
'0.928047066206695',
'0.0171322432434142',
1,
0
],
'id' => 444,
'r' => 6,
'cmt' => 'OPT s(0) optbs_spline_Re500000_N4_uag8814320.dat-s17_n17s0_nsopt-Re500000-N4.dat (opt90.8667166659173>71.4626960300354) l1 Foil spline 17pts Re500000 N4',
'Re' => 500000,
'sym' => '8.455684584572',
'Top_Xtr' => '0.651304328829135',
'good' => '81.478773599522',
'Foil_fn' => 'opt_spline_uag8814320_nsopt-Re500000-N4.dat',
'Bot_Xtr' => 1,
'alpha' => '3.3'
}
'section' => [
{
's' => 0,
'speed' => 0,
'flow_extra' => 0,
'section_radius' => '0',
'foil' => {},
'chord' => 0,
'aoa' => 0,
'max_power_watts' => 0,
'u' => '0',
'rotational_speed' => '0',
'zero_aoa' => 90
},
{
'flow_extra' => '25.3905676859905',
'xtilt' => 0,
's' => 1,
'hedral' => 0,
'aoa' => '3.7',
'scimitar' => 0,
'liftamt' => 1,
'section_radius' => '0.004375',
'move_tail' => '-0.034',
'speed' => '32.3577971333193',
'max_power_watts' => '12441.3781661353',
'chord' => '0.068',
'foil' => {
'Cm' => '-0.223073513627423',
'diter' => 4,
'r' => 6,
'id' => 372,
'xy' => [
1,
0,
'0.946714574040677',
'0.0246246154433233',
'0.854728633619417',
'0.0520861376632106',
'0.691363149797408',
'0.083139805289639',
'0.513763139089403',
'0.0994572780664641',
'0.327229099655296',
'0.0965396658039321',
'0.155573716106985',
'0.0732076331438239',
'0.0401397957317501',
'0.0350828038972155',
0,
0,
'0.0290164970646396',
'-0.00467510840254184',
'0.113543374367374',
'0.00726529952199307',
'0.264712879181914',
'0.0286489813907327',
'0.449425033656063',
'0.045123748387568',
'0.653083287629906',
'0.0478893590671292',
'0.83008632648885',
'0.0361652425378873',
'0.943258507857798',
'0.0177231938298917',
1,
0
],
'CDpres' => '0.00668',
'clcd' => '92.6901757815957',
'Foil_fn' => 'optbs_spline_e61.dat-s17_n17s0_nsopt-Re150000-N3.dat',
'good' => '48.9105167934696',
'Bot_Xtr' => 1,
'alpha' => '3.7',
'sym' => '7.7370440369536',
'Top_Xtr' => '0.635352929190835',
'cmt' => 'OPT s(0) foildb2020_spline/spline_foil_e61.dat-s17_n17s0.dat (opt48.9105167863018>43.0448397946629) l1 Foil spline 17pts Re150000 N3',
'Re' => 150000,
'CD' => '0.014234676287868',
'CDf' => '0.00756',
'iver' => '1.2020052',
'src' => 'retestc_23.txt',
'CL' => '1.3194146473166',
'Cpmin' => '-1.42745484622521',
'CDp' => '0.00238529361870813',
'n' => 3,
'xver' => '6.991'
},
'manualaoa' => 0,
'zero_aoa' => '87.8497042929691',
'rotational_speed' => '1.21409466091855',
'u' => '0.00694444444444444'
},
{
'scimitar' => 0,
'liftamt' => 1,
'aoa' => '3.7',
'flow_extra' => '25.3905676859905',
'xtilt' => 0,
's' => 2,
'hedral' => 0,
'zero_aoa' => '81.4586171992175',
'manualaoa' => 0,
'rotational_speed' => '4.85637864367421',
'u' => '0.0277777777777778',
'max_power_watts' => '12441.3781661353',
'chord' => '0.068',
'foil' => {
'clcd' => '92.6901757815957',
'CDpres' => '0.00668',
'diter' => 4,
'Cm' => '-0.223073513627423',
'xy' => [
1,
0,
'0.946714574040677',
'0.0246246154433233',
'0.854728633619417',
'0.0520861376632106',
'0.691363149797408',
'0.083139805289639',
'0.513763139089403',
'0.0994572780664641',
'0.327229099655296',
'0.0965396658039321',
'0.155573716106985',
'0.0732076331438239',
'0.0401397957317501',
'0.0350828038972155',
0,
0,
'0.0290164970646396',
'-0.00467510840254184',
'0.113543374367374',
'0.00726529952199307',
'0.264712879181914',
'0.0286489813907327',
'0.449425033656063',
'0.045123748387568',
'0.653083287629906',
'0.0478893590671292',
'0.83008632648885',
'0.0361652425378873',
'0.943258507857798',
'0.0177231938298917',
1,
0
],
'id' => 372,
'r' => 6,
'cmt' => 'OPT s(0) foildb2020_spline/spline_foil_e61.dat-s17_n17s0.dat (opt48.9105167863018>43.0448397946629) l1 Foil spline 17pts Re150000 N3',
'Re' => 150000,
'sym' => '7.7370440369536',
'Top_Xtr' => '0.635352929190835',
'Foil_fn' => 'optbs_spline_e61.dat-s17_n17s0_nsopt-Re150000-N3.dat',
'Bot_Xtr' => 1,
'alpha' => '3.7',
'good' => '48.9105167934696',
'CDf' => '0.00756',
'CD' => '0.014234676287868',
'Cpmin' => '-1.42745484622521',
'src' => 'retestc_23.txt',
'CL' => '1.3194146473166',
'n' => 3,
'xver' => '6.991',
'CDp' => '0.00238529361870813',
'iver' => '1.2020052'
},
'section_radius' => '0.0175',
'move_tail' => '-0.034',
'speed' => '32.6976669352128'
},
{
'section_radius' => '0.039375',
'move_tail' => '-0.034',
'speed' => '34.1313507346943',
'max_power_watts' => '12441.3781661353',
'chord' => '0.068',
'foil' => {
'CDf' => '0.00756',
'CD' => '0.014234676287868',
'CDp' => '0.00238529361870813',
'xver' => '6.991',
'n' => 3,
'CL' => '1.3194146473166',
'src' => 'retestc_23.txt',
'Cpmin' => '-1.42745484622521',
'iver' => '1.2020052',
'CDpres' => '0.00668',
'clcd' => '92.6901757815957',
'id' => 372,
'r' => 6,
'xy' => [
1,
0,
'0.946714574040677',
'0.0246246154433233',
'0.854728633619417',
'0.0520861376632106',
'0.691363149797408',
'0.083139805289639',
'0.513763139089403',
'0.0994572780664641',
'0.327229099655296',
'0.0965396658039321',
'0.155573716106985',
'0.0732076331438239',
'0.0401397957317501',
'0.0350828038972155',
0,
0,
'0.0290164970646396',
'-0.00467510840254184',
'0.113543374367374',
'0.00726529952199307',
'0.264712879181914',
'0.0286489813907327',
'0.449425033656063',
'0.045123748387568',
'0.653083287629906',
'0.0478893590671292',
'0.83008632648885',
'0.0361652425378873',
'0.943258507857798',
'0.0177231938298917',
1,
0
],
'Cm' => '-0.223073513627423',
'diter' => 4,
'Re' => 150000,
'cmt' => 'OPT s(0) foildb2020_spline/spline_foil_e61.dat-s17_n17s0.dat (opt48.9105167863018>43.0448397946629) l1 Foil spline 17pts Re150000 N3',
'Foil_fn' => 'optbs_spline_e61.dat-s17_n17s0_nsopt-Re150000-N3.dat',
'alpha' => '3.7',
'Bot_Xtr' => 1,
'good' => '48.9105167934696',
'Top_Xtr' => '0.635352929190835',
'sym' => '7.7370440369536'
},
'zero_aoa' => '71.3285350759113',
'manualaoa' => 0,
'rotational_speed' => '10.926851948267',
'u' => '0.0625',
'flow_extra' => '25.3905676859905',
'xtilt' => 0,
'hedral' => 0,
's' => 3,
'aoa' => '3.7',
'scimitar' => 0,
'liftamt' => 1
},
{
'flow_extra' => '25.3905676859905',
'xtilt' => 0,
'hedral' => 0,
's' => 4,
'scimitar' => 0,
'liftamt' => 1,
'aoa' => '3.7',
'section_radius' => '0.07',
'move_tail' => '-0.034',
'speed' => '37.7213948041046',
'rotational_speed' => '19.4255145746968',
'manualaoa' => 0,
'zero_aoa' => '59.0043236164718',
'u' => '0.111111111111111',
'max_power_watts' => '12441.3781661353',
'chord' => '0.068',
'foil' => {
'CDf' => '0.00756',
'CD' => '0.014234676287868',
'Cpmin' => '-1.42745484622521',
'CL' => '1.3194146473166',
'src' => 'retestc_23.txt',
'n' => 3,
'xver' => '6.991',
'CDp' => '0.00238529361870813',
'iver' => '1.2020052',
'clcd' => '92.6901757815957',
'CDpres' => '0.00668',
'diter' => 4,
'Cm' => '-0.223073513627423',
'xy' => [
1,
0,
'0.946714574040677',
'0.0246246154433233',
'0.854728633619417',
'0.0520861376632106',
'0.691363149797408',
'0.083139805289639',
'0.513763139089403',
'0.0994572780664641',
'0.327229099655296',
'0.0965396658039321',
'0.155573716106985',
'0.0732076331438239',
'0.0401397957317501',
'0.0350828038972155',
0,
0,
'0.0290164970646396',
'-0.00467510840254184',
'0.113543374367374',
'0.00726529952199307',
'0.264712879181914',
'0.0286489813907327',
'0.449425033656063',
'0.045123748387568',
'0.653083287629906',
'0.0478893590671292',
'0.83008632648885',
'0.0361652425378873',
'0.943258507857798',
'0.0177231938298917',
1,
0
],
'r' => 6,
'id' => 372,
'cmt' => 'OPT s(0) foildb2020_spline/spline_foil_e61.dat-s17_n17s0.dat (opt48.9105167863018>43.0448397946629) l1 Foil spline 17pts Re150000 N3',
'Re' => 150000,
'Top_Xtr' => '0.635352929190835',
'sym' => '7.7370440369536',
'Bot_Xtr' => 1,
'Foil_fn' => 'optbs_spline_e61.dat-s17_n17s0_nsopt-Re150000-N3.dat',
'alpha' => '3.7',
'good' => '48.9105167934696'
}
},
{
'xtilt' => 0,
'hedral' => 0,
's' => 5,
'flow_extra' => '25.3905676859905',
'liftamt' => 1,
'scimitar' => 0,
'aoa' => '2.8',
'speed' => '44.3488349680091',
'section_radius' => '0.109375',
'move_tail' => '-0.0381764132553606',
'u' => '0.173611111111111',
'manualaoa' => 0,
'rotational_speed' => '30.3523665229638',
'zero_aoa' => '46.8115188999663',
'chord' => '0.0763528265107213',
'foil' => {
'Foil_fn' => 'opt_spline_e61_nsopt-Re275000-N4.dat',
'good' => '56.2072715534896',
'alpha' => '2.8',
'Bot_Xtr' => 1,
'Top_Xtr' => '0.680534095358465',
'sym' => '8.38584277034114',
'Re' => 225000,
'cmt' => 'OPT s(0) optbs_spline_Re275000_N4_e61.dat-s17_n17s0_nsopt-Re275000-N4.dat (opt75.5452262403251>67.1718747466489) l1 Foil spline 17pts Re275000 N4',
'r' => 6,
'id' => 396,
'xy' => [
1,
0,
'0.946714574040677',
'0.0260556482335768',
'0.854728633619417',
'0.0551130670008592',
'0.691555011261055',
'0.0878645417414586',
'0.516159070249535',
'0.105059605208279',
'0.323702591682963',
'0.101358620191483',
'0.162837067505129',
'0.0764667171897211',
'0.0484320448643716',
'0.0366145511645788',
0,
0,
'0.0290628904959806',
'-0.00481324656358515',
'0.112660630004776',
'0.00753420185591148',
'0.292228965625459',
'0.032345828539994',
'0.453875285172211',
'0.0465459935519156',
'0.648361212442096',
'0.0495722905966673',
'0.83008632648885',
'0.0370300098723954',
'0.943278981389669',
'0.0180299268156232',
1,
0
],
'Cm' => '-0.2421301073138',
'diter' => 3,
'CDpres' => '0.00494',
'clcd' => '111.019960375868',
'iver' => '1.2020052',
'CDp' => '0.00146402123747234',
'n' => 3,
'xver' => '6.991',
'CL' => '1.28687554421646',
'src' => 'retest_97.txt',
'Cpmin' => '-1.19593006167197',
'CD' => '0.0115913889705926',
'CDf' => '0.00666'
},
'max_power_watts' => '12441.3781661353'
},
{
'xtilt' => 0,
'hedral' => 0,
's' => 6,
'flow_extra' => '25.3905676859905',
'aoa' => '1.6',
'liftamt' => 1,
'scimitar' => 0,
'speed' => '54.368101911552',
'section_radius' => '0.1575',
'move_tail' => '-0.0399752545977169',
'chord' => '0.0799505091954338',
'foil' => {
'clcd' => '118.217490119225',
'CDpres' => '0.00305',
'xy' => [
1,
0,
'0.946714574040677',
'0.0223621236083683',
'0.854728633619417',
'0.0473005010530232',
'0.691363149797408',
'0.0755009801855273',
'0.513763139089403',
'0.0903192153799569',
'0.327229099655296',
'0.08766967121931',
'0.155573716106985',
'0.0664813688240622',
'0.0401397957317501',
'0.0318594212804405',
0,
0,
'0.0290164970646396',
'-0.00419518862905253',
'0.113543374367374',
'0.00651948560695504',
'0.264712879181914',
'0.0257080415288325',
'0.449425033656063',
'0.0404916035813907',
'0.653083287629906',
'0.0429733125550207',
'0.83008632648885',
'0.032452726482103',
'0.943258507857798',
'0.015903832558242',
1,
0
],
'r' => 6,
'id' => 408,
'diter' => 3,
'Cm' => '-0.216581298953389',
'Re' => 275000,
'cmt' => 'OPT s(0) ../foildb2020_spline/spline_foil_e61.dat-s17_n17s0.dat (opt64.8062967518512>61.9736441951258) l1 Foil spline 17pts Re500000 N8',
'sym' => '6.33573166885975',
'Top_Xtr' => '0.709309373884831',
'Bot_Xtr' => 1,
'alpha' => '1.6',
'good' => '62.2441466730698',
'Foil_fn' => 'optbs-spline_foil_e61.dat-s17_n17s0-Re500000-N8-sq1.dat',
'CDf' => '0.00599',
'CD' => '0.00903568446362737',
'xver' => '6.991',
'n' => 3,
'CDp' => '0.000942084288359758',
'Cpmin' => '-0.962053314848102',
'src' => 'retest_142.txt',
'CL' => '1.0681759387993',
'iver' => '1.2020052'
},
'max_power_watts' => '12441.3781661353',
'u' => '0.25',
'rotational_speed' => '43.7074077930679',
'manualaoa' => 0,
'zero_aoa' => '36.4942662532566'
},
{
'u' => '0.340277777777778',
'rotational_speed' => '59.4906383850091',
'manualaoa' => 0,
'zero_aoa' => '28.5254557024781',
'chord' => '0.080982182032523',
'foil' => {
'Cpmin' => '-1.41743602842382',
'src' => 'retest_105.txt',
'CL' => '1.4063309867754',
'n' => 3,
'xver' => '6.991',
'CDp' => '0.00217293983362531',
'iver' => '1.2020052',
'CDf' => '0.00553',
'CD' => '0.0110664225240897',
'cmt' => 'OPT s(0) optbs_spline_Re500000_N4_uag8814320.dat-s17_n17s0_nsopt-Re500000-N4.dat (opt90.8667166659173>71.4626960300354) l1 Foil spline 17pts Re500000 N4',
'Re' => 350000,
'sym' => '8.455684584572',
'Top_Xtr' => '0.646694568543603',
'Foil_fn' => 'opt_spline_uag8814320_nsopt-Re500000-N4.dat',
'alpha' => '4.2',
'Bot_Xtr' => 1,
'good' => '72.15391188971',
'clcd' => '127.080904756172',
'CDpres' => '0.00554',
'diter' => 3,
'Cm' => '-0.223765678670715',
'xy' => [
1,
0,
'0.976945036273593',
'0.00939462486134211',
'0.851587448517823',
'0.0496390744878245',
'0.698540191794841',
'0.104268363253013',
'0.518781216857077',
'0.131891298315886',
'0.327066076343497',
'0.126506814697001',
'0.150680323221142',
'0.0905868417616979',
'0.0395735702112627',
'0.0422057839630234',
0,
0,
'0.0572978334471142',
'-0.00364596812263481',
'0.162662166368623',
'0.00417792129992324',
'0.31244663898479',
'0.0140082041081467',
'0.472099215454883',
'0.0216480338049078',
'0.578263018146512',
'0.0257475498551808',
'0.759908593228447',
'0.0266643873940281',
'0.928047066206695',
'0.0171322432434142',
1,
0
],
'id' => 420,
'r' => 6
},
'max_power_watts' => '12441.3781661353',
'speed' => '67.7103320397359',
'section_radius' => '0.214375',
'move_tail' => '-0.0404910910162615',
'liftamt' => 1,
'scimitar' => 0,
'aoa' => '4.2',
'xtilt' => 0,
'hedral' => 0,
's' => 7,
'flow_extra' => '25.3905676859905'
},
{
'chord' => '0.0801993830329459',
'foil' => {
'CD' => '0.0101633985049173',
'CDf' => '0.00533',
'CDp' => '0.00184813590237463',
'n' => 3,
'xver' => '6.991',
'CL' => '1.38749385928828',
'src' => 'retestc_2.txt',
'Cpmin' => '-1.40228951594593',
'iver' => '1.2020052',
'CDpres' => '0.00483',
'clcd' => '136.51869092971',
'r' => 6,
'id' => 432,
'xy' => [
1,
0,
'0.976945036273593',
'0.00939462486134211',
'0.851587448517823',
'0.0496390744878245',
'0.698540191794841',
'0.104268363253013',
'0.518781216857077',
'0.131891298315886',
'0.327066076343497',
'0.126506814697001',
'0.150680323221142',
'0.0905868417616979',
'0.0395735702112627',
'0.0422057839630234',
0,
0,
'0.0572978334471142',
'-0.00364596812263481',
'0.162662166368623',
'0.00417792129992324',
'0.31244663898479',
'0.0140082041081467',
'0.472099215454883',
'0.0216480338049078',
'0.578263018146512',
'0.0257475498551808',
'0.759908593228447',
'0.0266643873940281',
'0.928047066206695',
'0.0171322432434142',
1,
0
],
'Cm' => '-0.226090452157689',
'diter' => 3,
'Re' => 400000,
'cmt' => 'OPT s(0) optbs_spline_Re500000_N4_uag8814320.dat-s17_n17s0_nsopt-Re500000-N4.dat (opt90.8667166659173>71.4626960300354) l1 Foil spline 17pts Re500000 N4',
'good' => '76.3652666263628',
'Foil_fn' => 'opt_spline_uag8814320_nsopt-Re500000-N4.dat',
'alpha' => '3.9',
'Bot_Xtr' => 1,
'Top_Xtr' => '0.64776972987106',
'sym' => '8.455684584572'
},
'max_power_watts' => '12441.3781661353',
'u' => '0.444444444444444',
'manualaoa' => 0,
'rotational_speed' => '77.7020582987874',
'zero_aoa' => '22.5941970042176',
'speed' => '84.1615284636842',
'section_radius' => '0.28',
'move_tail' => '-0.040099691516473',
'aoa' => '3.9',
'liftamt' => 1,
'scimitar' => 0,
'xtilt' => 0,
'hedral' => 0,
's' => 8,
'flow_extra' => '25.3905676859905'
},
{
'flow_extra' => '25.3905676859905',
'hedral' => 0,
's' => 9,
'xtilt' => 0,
'aoa' => '3.3',
'scimitar' => 0,
'liftamt' => 1,
'move_tail' => '-0.03838428169042',
'section_radius' => '0.354375',
'speed' => '103.521189052881',
'max_power_watts' => '12441.3781661353',
'foil' => {
'clcd' => '151.377131465261',
'CDpres' => '0.00385',
'diter' => 3,
'Cm' => '-0.228915601237377',
'xy' => [
1,
0,
'0.976945036273593',
'0.00939462486134211',
'0.851587448517823',
'0.0496390744878245',
'0.698540191794841',
'0.104268363253013',
'0.518781216857077',
'0.131891298315886',
'0.327066076343497',
'0.126506814697001',
'0.150680323221142',
'0.0905868417616979',
'0.0395735702112627',
'0.0422057839630234',
0,
0,
'0.0572978334471142',
'-0.00364596812263481',
'0.162662166368623',
'0.00417792129992324',
'0.31244663898479',
'0.0140082041081467',
'0.472099215454883',
'0.0216480338049078',
'0.578263018146512',
'0.0257475498551808',
'0.759908593228447',
'0.0266643873940281',
'0.928047066206695',
'0.0171322432434142',
1,
0
],
'r' => 6,
'id' => 444,
'cmt' => 'OPT s(0) optbs_spline_Re500000_N4_uag8814320.dat-s17_n17s0_nsopt-Re500000-N4.dat (opt90.8667166659173>71.4626960300354) l1 Foil spline 17pts Re500000 N4',
'Re' => 500000,
'sym' => '8.455684584572',
'Top_Xtr' => '0.651304328829135',
'Foil_fn' => 'opt_spline_uag8814320_nsopt-Re500000-N4.dat',
'Bot_Xtr' => 1,
'good' => '81.478773599522',
'alpha' => '3.3',
'CD' => '0.00884978018634026',
'CDf' => '0.005',
'Cpmin' => '-1.36452160346992',
'src' => 'retestc_7.txt',
'CL' => '1.33965433870629',
'n' => 3,
'xver' => '6.991',
'CDp' => '0.00143473501628707',
'iver' => '1.2020052'
},
'chord' => '0.0767685633808401',
'manualaoa' => 0,
'rotational_speed' => '98.3416675344028',
'zero_aoa' => '18.20100791921',
'u' => '0.5625'
},
{
'liftamt' => 1,
'scimitar' => 0,
'aoa' => '3.2',
'xtilt' => 0,
'hedral' => 0,
's' => 10,
'flow_extra' => '25.3905676859905',
'u' => '0.694444444444445',
'zero_aoa' => '14.913419516814',
'manualaoa' => 0,
'rotational_speed' => '121.409466091855',
'chord' => '0.0697949740902727',
'foil' => {
'Cpmin' => '-1.36371990221532',
'CL' => '1.33918512298929',
'src' => 'retestc_4.txt',
'n' => 3,
'xver' => '6.991',
'CDp' => '0.00119558206670186',
'iver' => '1.2020052',
'CD' => '0.00819400687973172',
'CDf' => '0.00476',
'cmt' => 'OPT s(0) optbs_spline_Re500000_N4_uag8814320.dat-s17_n17s0_nsopt-Re500000-N4.dat (opt90.8667166659173>71.4626960300354) l1 Foil spline 17pts Re500000 N4',
'Re' => 620000,
'Top_Xtr' => '0.63980650954827',
'sym' => '8.455684584572',
'good' => '83.8091083549883',
'Foil_fn' => 'opt_spline_uag8814320_nsopt-Re500000-N4.dat',
'alpha' => '3.2',
'Bot_Xtr' => '0.957557098624352',
'clcd' => '163.434708152593',
'CDpres' => '0.00344',
'diter' => 3,
'Cm' => '-0.230934860507051',
'xy' => [
1,
0,
'0.976945036273593',
'0.00939462486134211',
'0.851587448517823',
'0.0496390744878245',
'0.698540191794841',
'0.104268363253013',
'0.518781216857077',
'0.131891298315886',
'0.327066076343497',
'0.126506814697001',
'0.150680323221142',
'0.0905868417616979',
'0.0395735702112627',
'0.0422057839630234',
0,
0,
'0.0572978334471142',
'-0.00364596812263481',
'0.162662166368623',
'0.00417792129992324',
'0.31244663898479',
'0.0140082041081467',
'0.472099215454883',
'0.0216480338049078',
'0.578263018146512',
'0.0257475498551808',
'0.759908593228447',
'0.0266643873940281',
'0.928047066206695',
'0.0171322432434142',
1,
0
],
'id' => 456,
'r' => 6
},
'max_power_watts' => '12441.3781661353',
'speed' => '125.641599266265',
'section_radius' => '0.4375',
'move_tail' => '-0.0348974870451364'
},
{
'xtilt' => 0,
'hedral' => 0,
's' => 11,
'flow_extra' => '25.3905676859905',
'liftamt' => 1,
'scimitar' => 0,
'aoa' => '3.2',
'speed' => '150.421957891604',
'section_radius' => '0.529375',
'move_tail' => '-0.0291883730888965',
'u' => '0.840277777777778',
'rotational_speed' => '146.905453971145',
'manualaoa' => 0,
'zero_aoa' => '12.4133007539681',
'chord' => '0.058376746177793',
'foil' => {
'Foil_fn' => 'opt_spline_uag8814320_nsopt-Re500000-N4.dat',
'alpha' => '3.2',
'good' => '83.8091083549883',
'Bot_Xtr' => '0.957557098624352',
'sym' => '8.455684584572',
'Top_Xtr' => '0.63980650954827',
'cmt' => 'OPT s(0) optbs_spline_Re500000_N4_uag8814320.dat-s17_n17s0_nsopt-Re500000-N4.dat (opt90.8667166659173>71.4626960300354) l1 Foil spline 17pts Re500000 N4',
'Re' => 620000,
'Cm' => '-0.230934860507051',
'diter' => 3,
'r' => 6,
'id' => 456,
'xy' => [
1,
0,
'0.976945036273593',
'0.00939462486134211',
'0.851587448517823',
'0.0496390744878245',
'0.698540191794841',
'0.104268363253013',
'0.518781216857077',
'0.131891298315886',
'0.327066076343497',
'0.126506814697001',
'0.150680323221142',
'0.0905868417616979',
'0.0395735702112627',
'0.0422057839630234',
0,
0,
'0.0572978334471142',
'-0.00364596812263481',
'0.162662166368623',
'0.00417792129992324',
'0.31244663898479',
'0.0140082041081467',
'0.472099215454883',
'0.0216480338049078',
'0.578263018146512',
'0.0257475498551808',
'0.759908593228447',
'0.0266643873940281',
'0.928047066206695',
'0.0171322432434142',
1,
0
],
'CDpres' => '0.00344',
'clcd' => '163.434708152593',
'iver' => '1.2020052',
'src' => 'retestc_4.txt',
'CL' => '1.33918512298929',
'Cpmin' => '-1.36371990221532',
'CDp' => '0.00119558206670186',
'xver' => '6.991',
'n' => 3,
'CDf' => '0.00476',
'CD' => '0.00819400687973172'
},
'max_power_watts' => '12441.3781661353'
},
{
'flow_extra' => '25.3905676859905',
'hedral' => 0,
's' => 12,
'xtilt' => 0,
'scimitar' => 0,
'liftamt' => 1,
'aoa' => '3.3',
'move_tail' => '-0.0208416153242169',
'section_radius' => '0.63',
'speed' => '177.794693242818',
'manualaoa' => 0,
'rotational_speed' => '174.829631172272',
'zero_aoa' => '10.4785342765095',
'u' => '1',
'max_power_watts' => '12441.3781661353',
'foil' => {
'CDpres' => '0.00385',
'clcd' => '151.377131465261',
'r' => 6,
'id' => 444,
'xy' => [
1,
0,
'0.976945036273593',
'0.00939462486134211',
'0.851587448517823',
'0.0496390744878245',
'0.698540191794841',
'0.104268363253013',
'0.518781216857077',
'0.131891298315886',
'0.327066076343497',
'0.126506814697001',
'0.150680323221142',
'0.0905868417616979',
'0.0395735702112627',
'0.0422057839630234',
0,
0,
'0.0572978334471142',
'-0.00364596812263481',
'0.162662166368623',
'0.00417792129992324',
'0.31244663898479',
'0.0140082041081467',
'0.472099215454883',
'0.0216480338049078',
'0.578263018146512',
'0.0257475498551808',
'0.759908593228447',
'0.0266643873940281',
'0.928047066206695',
'0.0171322432434142',
1,
0
],
'Cm' => '-0.228915601237377',
'diter' => 3,
'Re' => 500000,
'cmt' => 'OPT s(0) optbs_spline_Re500000_N4_uag8814320.dat-s17_n17s0_nsopt-Re500000-N4.dat (opt90.8667166659173>71.4626960300354) l1 Foil spline 17pts Re500000 N4',
'Foil_fn' => 'opt_spline_uag8814320_nsopt-Re500000-N4.dat',
'alpha' => '3.3',
'Bot_Xtr' => 1,
'good' => '81.478773599522',
'sym' => '8.455684584572',
'Top_Xtr' => '0.651304328829135',
'CD' => '0.00884978018634026',
'CDf' => '0.005',
'CDp' => '0.00143473501628707',
'xver' => '6.991',
'n' => 3,
'CL' => '1.33965433870629',
'src' => 'retestc_7.txt',
'Cpmin' => '-1.36452160346992',
'iver' => '1.2020052'
},
'chord' => '0.0416832306484338'
}
],
}
The above created this: https://a360.co/36kQfWH
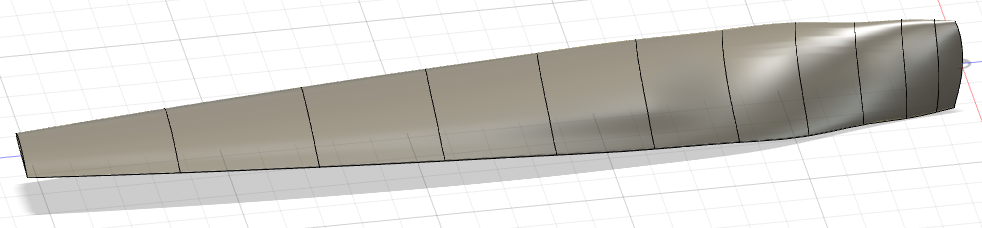
from this:

(here's more info about it:
)
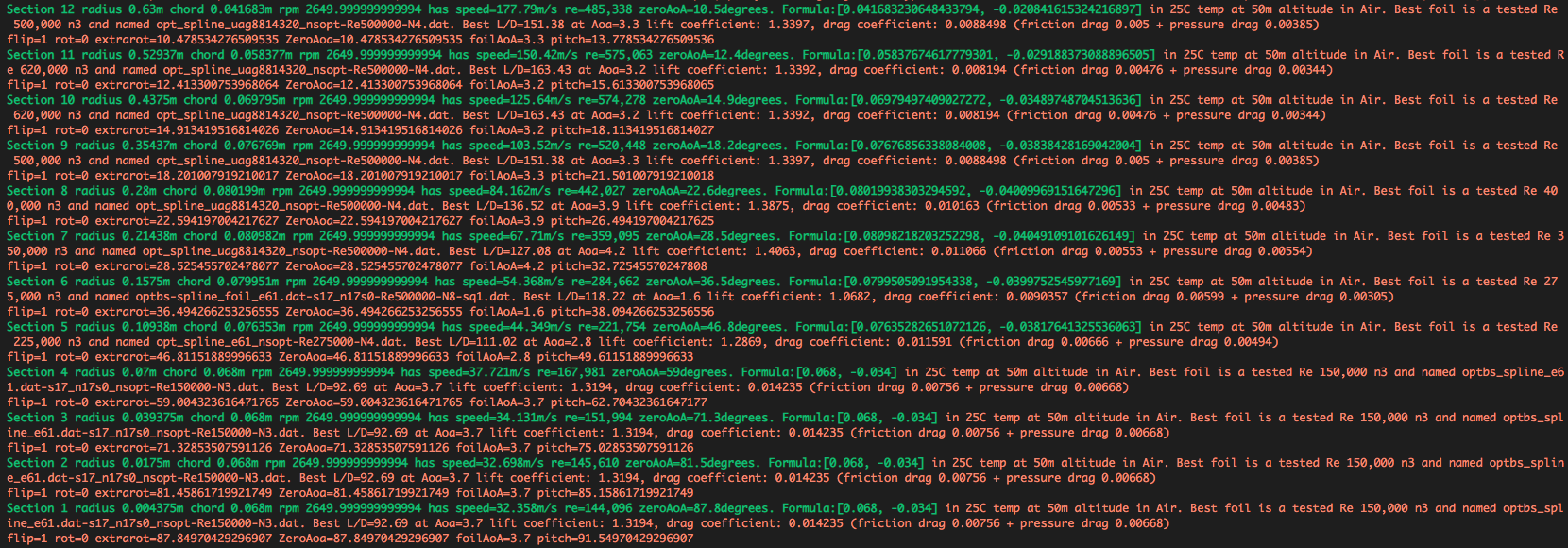
Here's an example boat-prop formula:-